Identifying and fixing deadlocks in Java
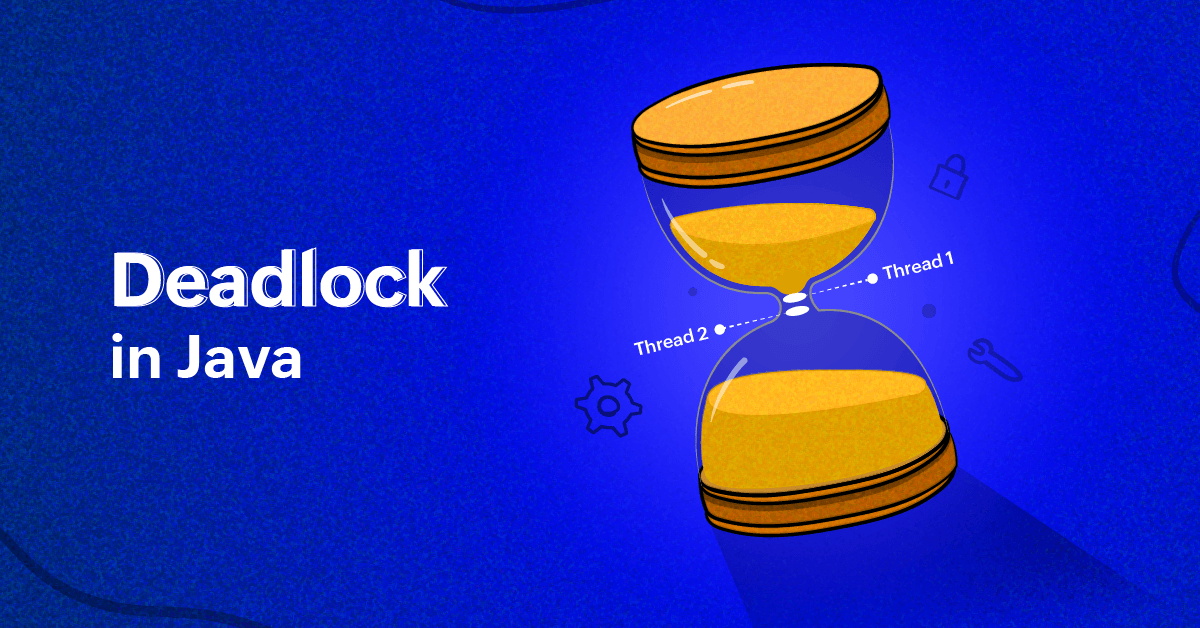
Why deadlock occurs
Deadlocks are common in multi-threaded applications and can be caused by improper handling of shared resources such as:
- Inadequate synchronization: When the resources are accessed by the threads with improper synchronization, it can lead to an unpredictable behavior called a race condition. This is when two threads aim for the same resource simultaneously.
- Incorrect resource release order: A thread might not release a resource when it finishes its work, causing other threads waiting for that resource to block indefinitely.
- Database deadlocks: In applications interacting with databases, if two threads are accessing database connections without proper locking or if they hold onto locks longer than necessary, a deadlock can occur.
- I/O blocking: When I/O operations (e.g., network calls, file reads) are not properly handled in a thread-safe manner, it can lead to deadlocks as threads wait for I/O completion.
Why fixing deadlocks is crucial
- Performance degradation: The application will stop processing new requests, causing slow response times.
- User dissatisfaction: End-users may experience long wait times or unresponsiveness.
- Data loss: In some cases, incomplete transactions can lead to data inconsistency or loss (e.g., database rollbacks due to deadlocks).
- System resource exhaustion: High CPU usage due to thread blocking can strain system resources.
Best practices for identifying and fixing deadlocks
- Monitor and track resources:
Use tools like a thread dump analyzer to analyze the state of threads periodically and identify potential deadlocks.
Look for patterns where certain resources are being acquired by multiple threads without release.
- Implement proper resource management:
Ensure that all shared resources (e.g., database connections, locks) are released as soon as possible after use.
Use try-with-resourcesor finally blocks to automatically release resources when they are no longer needed.
- Detect deadlocks proactively:
Set timeouts for acquiring resources (e.g., database connections).
Use locks with expiration policies.
Implement deadlock detection code in your application.
- Use thread-safe data structures and algorithms:
Use thread-safe data structures like ConcurrentHashMap or BlockingQueue to avoid contentions that could lead to deadlocks.
Use algorithms that are designed for concurrent environments, such as using a fair scheduler for resource allocation.
Examples of deadlocks
- Database connections:
Imagine a web application processing orders simultaneously. If two threads try to update the status of an order and both require exclusive access to a database table, without proper synchronization, they might deadlock, causing the entire system to freeze.
- Resource allocation in web applications:
In a system where each request requires allocating a temporary file or a new database connection, improper resource management can lead to contention and potential deadlocks as threads compete for limited resources.
- Contention vs. deadlock:
Contention occurs when multiple threads try to access the same resource without proper synchronization, leading to performance issues but not system failure.
Deadlock, however, involves mutual blocking, resulting in indefinite waiting and application crash.
Preventing deadlocks before they occur
- Design with resource constraints in mind:
Identify all shared resources and ensure they are not overused or mismanaged.
Design the application so that resources are released promptly to avoid blocking other threads.
- Simplify resource access:
Minimize the number of shared resources if possible.
Avoid complex interdependencies between threads that could lead to mutual waits.
- Implement synchronization correctly:
Use appropriate synchronization mechanisms (e.g., synchronized, lock) to ensure only one thread can access a resource at a time.
Avoid using wait() and notify() without proper ordering, as this can lead to deadlocks if not handled correctly.
- Test for deadlocks during development:
- Use tools like JMeter or Gatling for performance testing to simulate high-concurrency scenarios and identify potential deadlocks.
Conduct manual thread analysis to ensure that the application does not hold onto resources unnecessarily.
Role of thread dump analyzer and APM Tools in fixing Deadlocks
A thread dump analyzer is a tool that can analyze the stack traces from a Java thread dump to identify deadlocks, stuck threads, and other issues. It provides insights into:
Identifying deadlock scenarios: By analyzing the call stacks of blocked threads, you can determine which resources are causing the deadlock.
Detecting resource contention: The tool can highlight if multiple threads are competing for the same resource, leading to potential deadlocks.
- Real-time monitoring: Continuously monitor thread states and resource usage in your Java application.
- Alerting on deadlocks: Set up alerts when a deadlock is detected or when threads are stuck for an extended period.
- Root cause analysis: Provide detailed insights into the root cause of deadlocks by analyzing stack traces, resource contention, and transaction logs.
- Guided resolution: Offer recommendations to resolve deadlocks, such as releasing resources prematurely or reorganizing thread access patterns.
By combining a thread dump analyzer with an APM tool, you can proactively detect and fix deadlocks before they escalate into application failure. This ensures better performance, reliability, and user satisfaction for your application.