Understanding script errors and how to resolve them
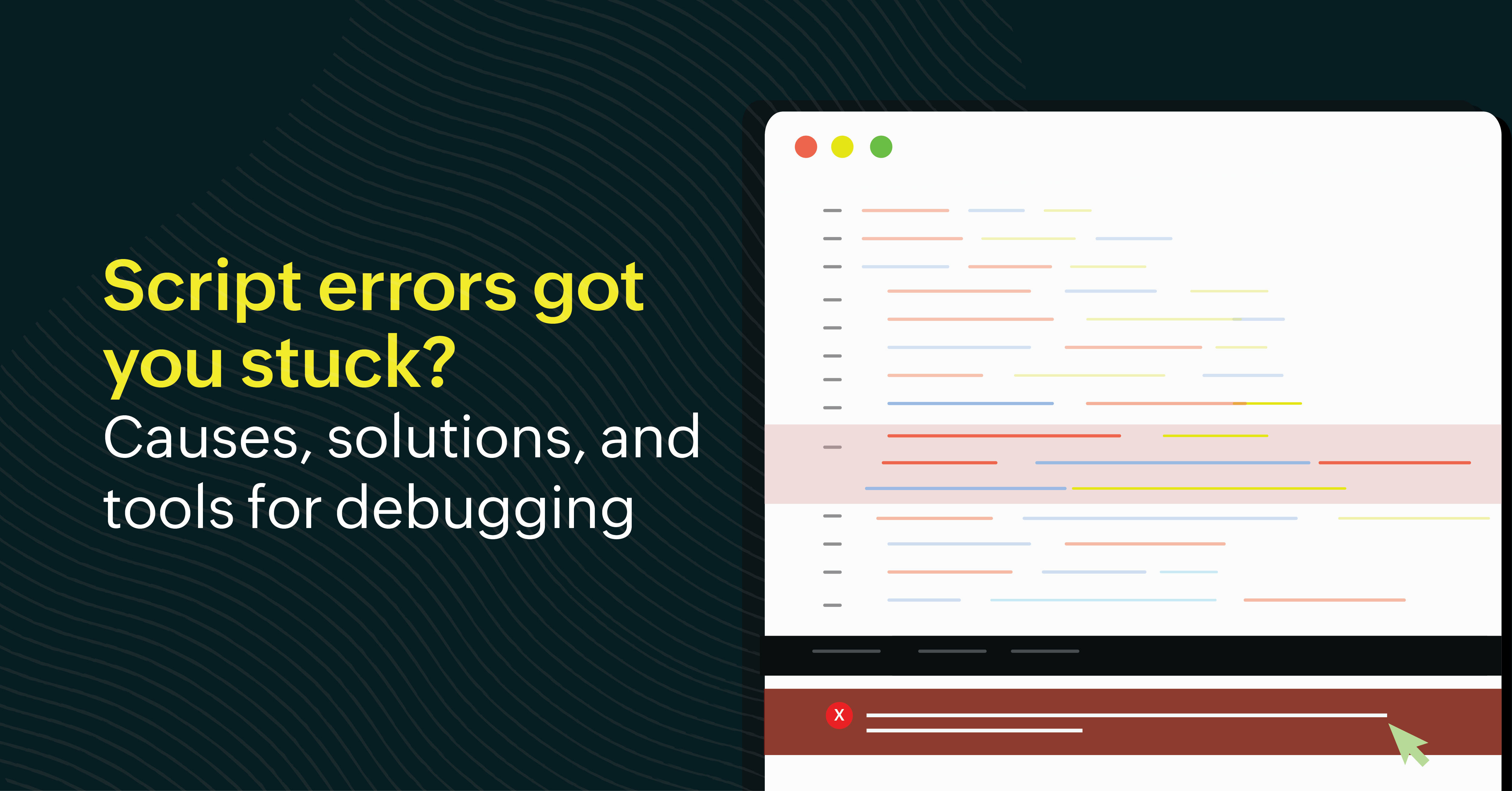
Nothing is more frustrating for users than navigating a website only to encounter errors that interrupt their journey. Script errors, a common issue in web applications, are particularly challenging because they provide minimal information for diagnosis, showing only a cryptic "Script error" message in the browser console.
Let's explore what script errors are, their common causes, and how you can resolve them effectively.
What is a script error?
A script error happens when a JavaScript exception occurs in a script hosted in a different origin than the current webpage. The origin could be a different domain, subdomain, port, or even a different protocol from the current page. Due to the same-origin policy enforced by browsers for security, these exceptions are restricted in the details they reveal, resulting in only a generic “Script error” message in the browser console.
The same-origin policy helps prevent cross-site request forgery and other security issues by limiting the interactions between different origins, such as data access and manipulation across domains. While this keeps user data safer, it can also make cross-origin debugging more difficult because browsers are unable to display specifics, such as the file, line number, or stack trace, for exceptions from third-party scripts.
Common causes of script errors
Cross-origin resource sharing issues
Modern browsers enforce cross-origin resource sharing (CORS) policies to restrict resources requested from a different origin. This is a standard security measure to prevent malicious scripts from interacting with or accessing data across domains without permission. However, if a script is loaded from a third-party origin (such as a CDN or an analytics provider) without proper CORS headers, the browser will block detailed error reporting, resulting in a script error. For example, loading a JavaScript file from https://zylker.example.com on your website, https://yourdomain.com, without CORS headers will trigger this error because the browser will restrict interactions with the third-party resource.
Third-party integration issues
Websites often rely on third-party scripts for services like analytics, ads, or social media features. If one of these scripts experiences an issue or if it is blocked by the browser (due to user privacy settings or ad blockers), a script error can occur. As third-party scripts run independently, their performance can be unpredictable, leading to potential errors when integrated into a site. For example, if a social media sharing plugin is blocked by a privacy extension, a script error could appear because the resource is failing to load as expected.
Browser incompatibility
Different browsers and their versions interpret JavaScript in varying ways, which can lead to errors, especially if the user’s browser is outdated or doesn’t support the latest JavaScript features. A feature that runs smoothly in one browser might trigger a script error in another, particularly if the feature relies on newer JavaScript capabilities.
How to resolve script errors
Here are some approaches to troubleshoot and resolve script errors effectively:
Configure CORS headers correctly
A controlled relaxation can be provided to instruct browsers to allow access to CORS specification through certain header instructions. Ensure that any third-party server serving JavaScript resources to your application includes the Access-Control-Allow-Origin header to allow cross-origin requests. The server should set this header to the domain of your application or to * if there are no restrictions (e.g., Access-Control-Allow-Origin: *). Adding the crossorigin attribute to your <script> tags in HTML enables full-stack trace visibility, which in turn facilitates more effective debugging of the the JavaScript errors.
Implement graceful fallbacks for third-party scripts
Whenever possible, design your application to work even if a third-party script fails. For instance, if an analytics script is unavailable, consider logging data locally and sending it when the script becomes available, ensuring your site remains functional without dependencies on external scripts. Also, incorporate error-handling mechanisms into your code, such as try...catch blocks, to capture and manage exceptions without affecting the user experience.
- try {// Code that might throw an error} catch (error) {console.error('Script error occurred:', error);}
Test browser compatibility
Simulate your application across different browsers and operating systems, allowing you to catch potential compatibility issues before deployment. Testing in a variety of environments helps ensure consistent performance across user devices.
Use a JavaScript error monitoring tool
Real user monitoring (RUM) tools capture detailed error reports, including the stack trace and metadata (such as the browser version, device, and application version). By implementing RUM monitoring tools, you can gain visibility into client-side errors and identify patterns in these errors to address them effectively.
Even with preventive measures, script errors are sometimes unavoidable, especially when dealing with third-party services. To stay proactive, Site24x7's RUM offers powerful capabilities to help you identify and troubleshoot these errors effectively.
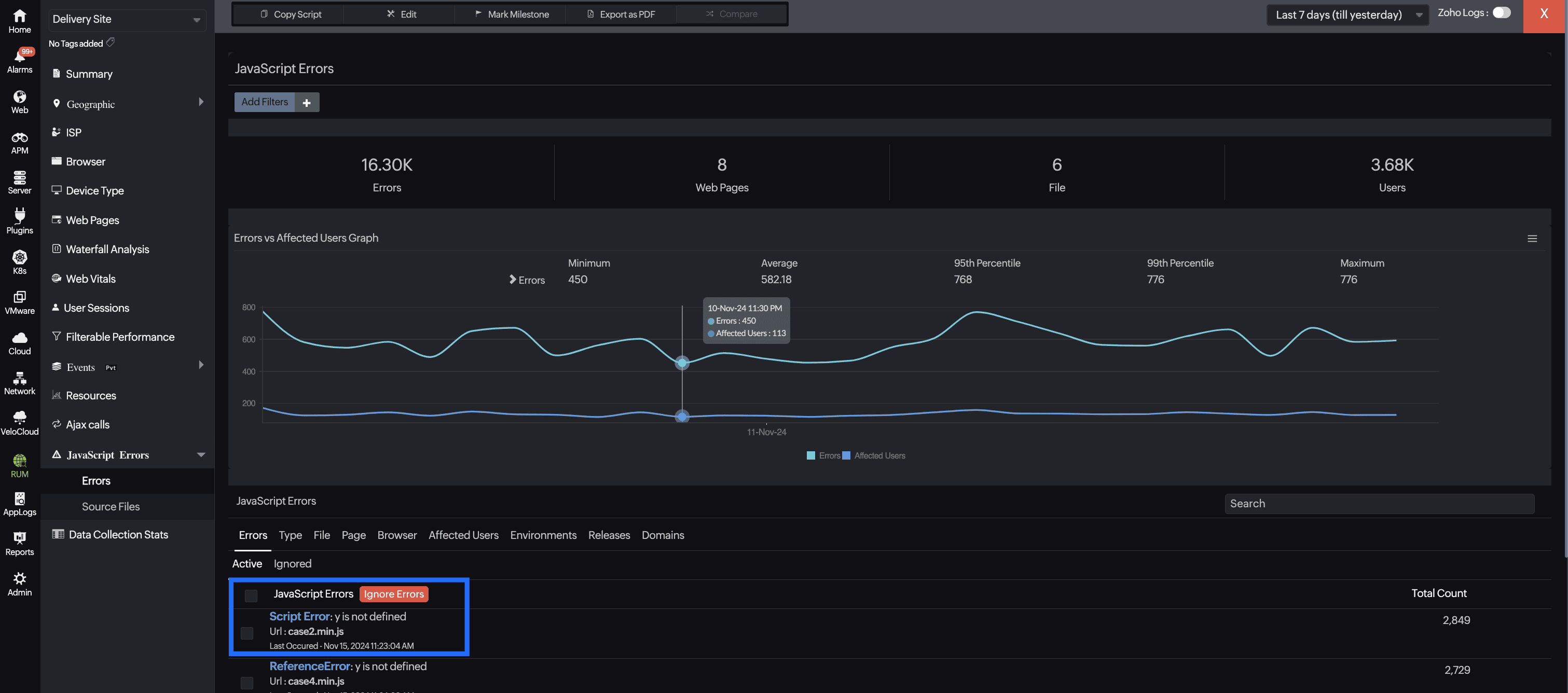
With RUM, you can:
- Track script errors in real time: Get detailed reports on client-side errors, including information on stack traces, timestamps, and affected pages.
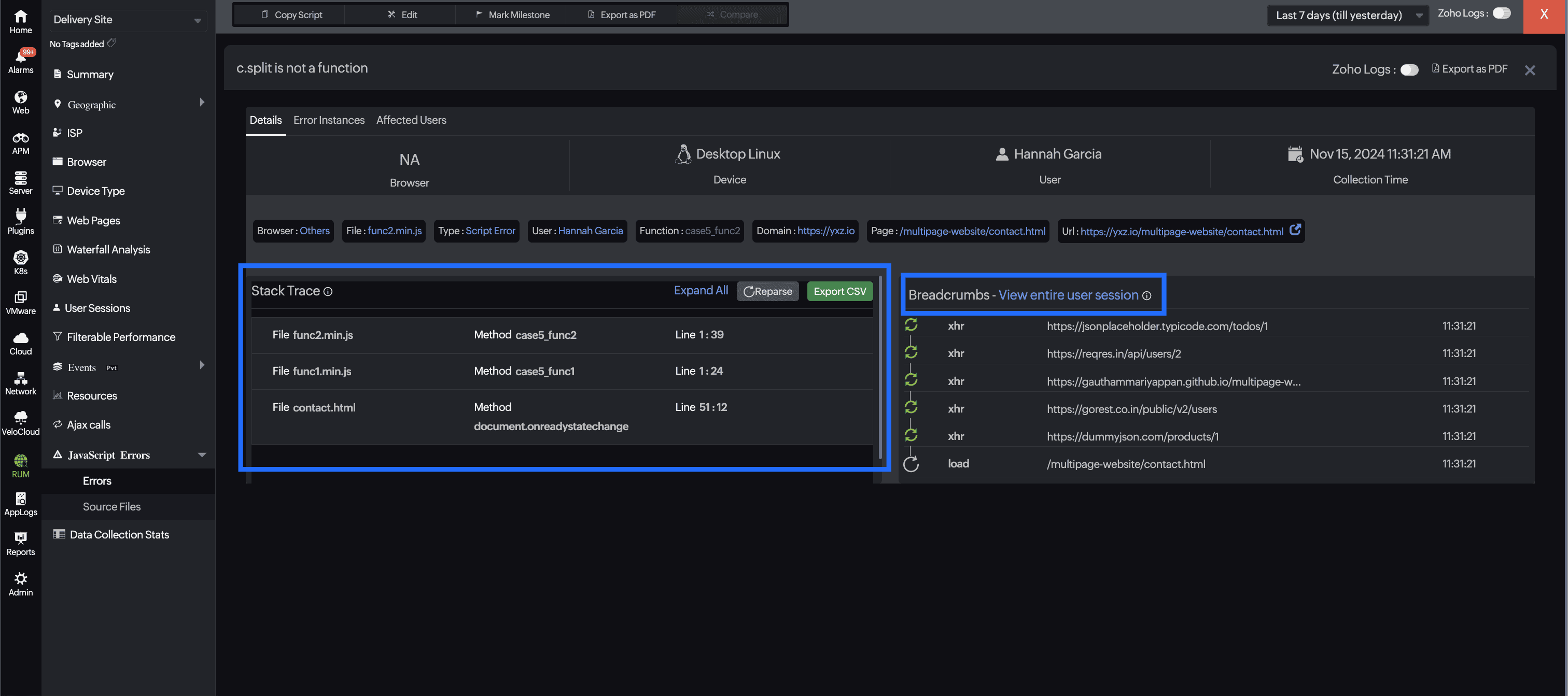
- Understand the impact on users: Use RUM to correlate script errors with specific users, enabling you to prioritize issues based on their impact on the user experience.
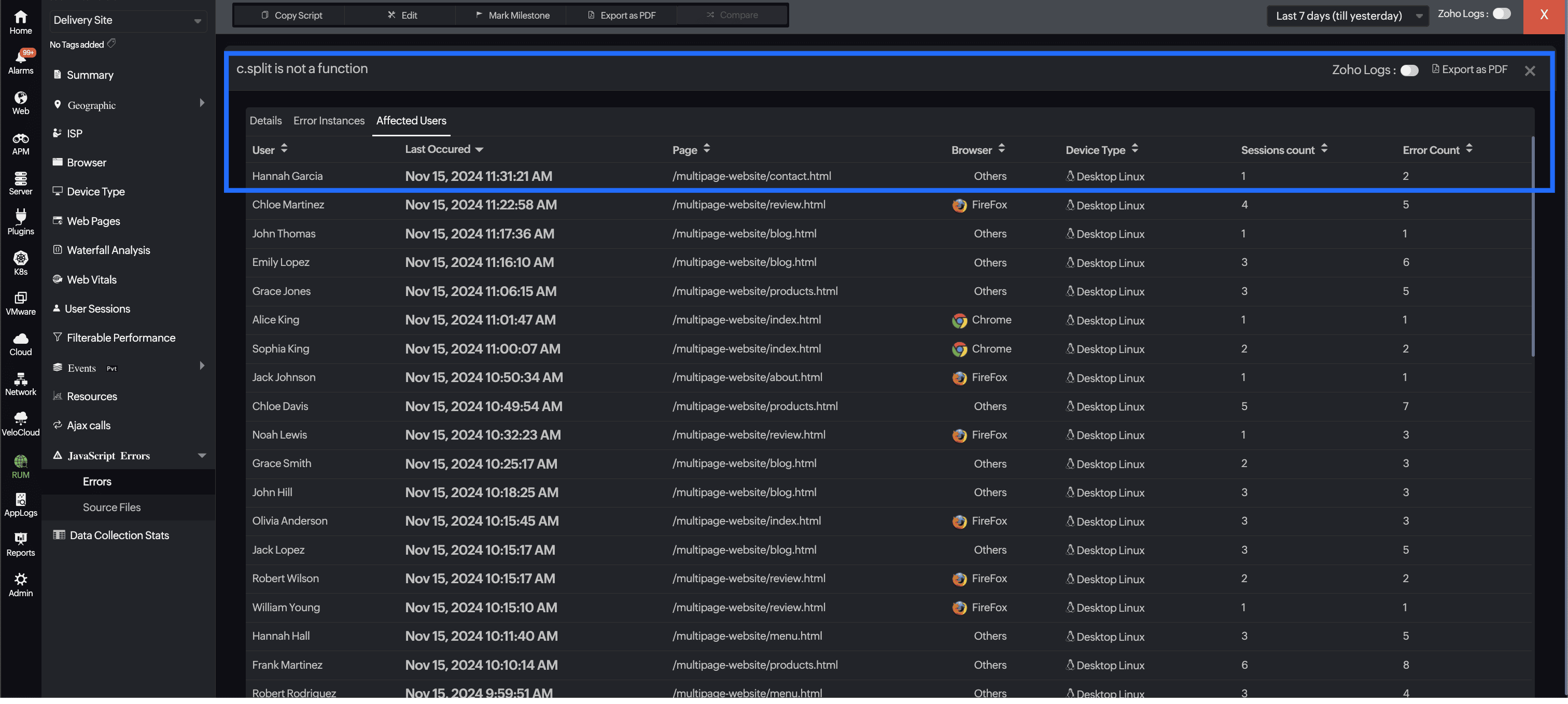
- Analyze trends: Identify recurring script errors and monitor their frequency to understand their root causes better.
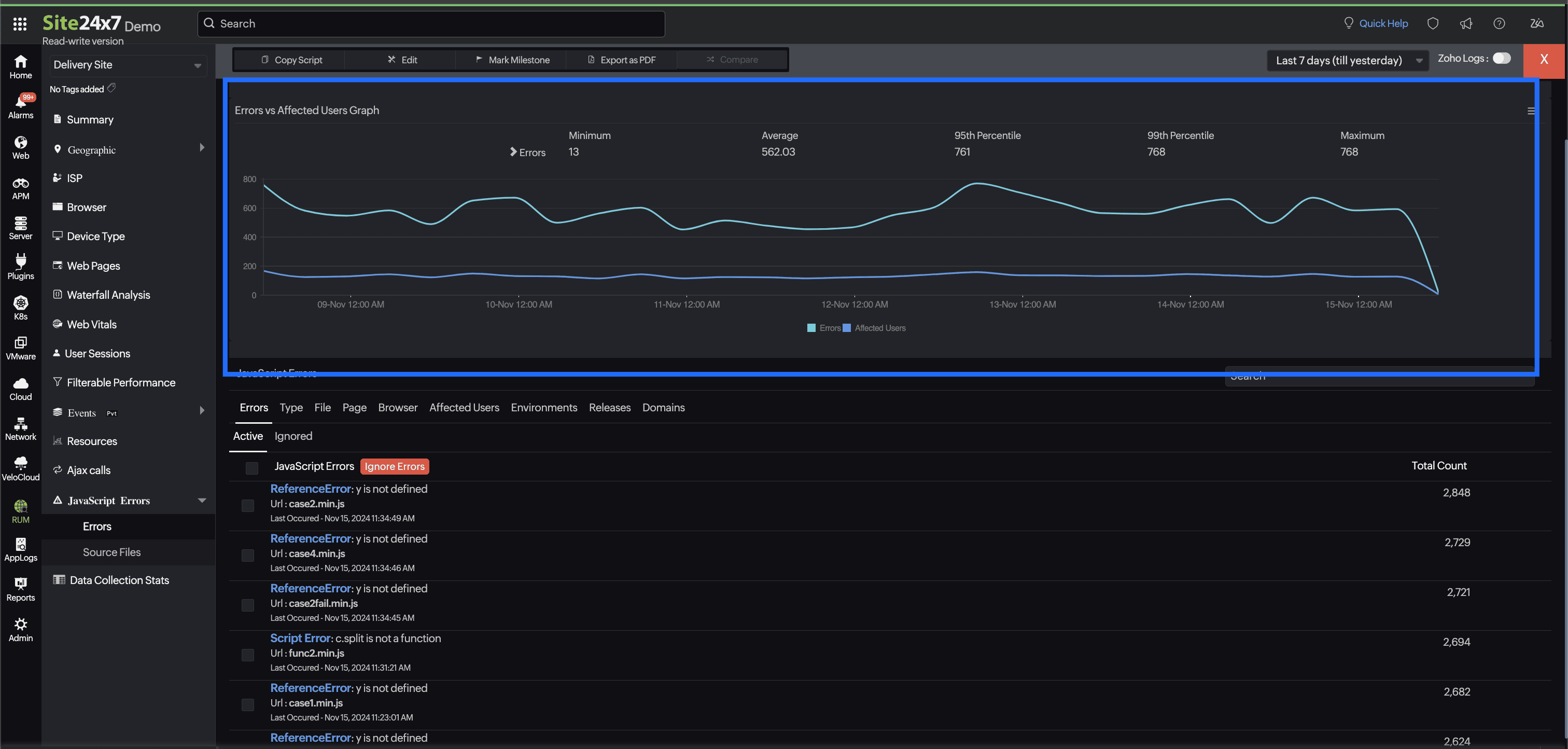
By using RUM tools, developers can quickly gain visibility into script errors, reduce the time spent troubleshooting, and ensure a smoother experience for their users.
Script errors disrupt the user experience and create challenges in debugging, especially when they arise from cross-origin issues. By understanding common causes like CORS misconfigurations, third-party integration issues, and browser incompatibility, you can effectively resolve these errors. For a more efficient approach to identifying and addressing script errors, Site24x7's RUM offers real-time tracking and insights, enabling you to maintain a robust, reliable application experience.
Comments (0)